In R, operators allow you to perform various tasks, from basic arithmetic operations to data manipulation and comparison tasks. There are different kinds of operators in R, which include arithmetic, logical, assignment, and comparison operators etc.
Assignment Operators in R
Assignment operators are used to assign values to variables in R. They include the assignment operator “<-” and “=” the right assignment operator ->. The assignment operator “<-” is simply used to assign a value to a variable. For instance, if we have a variable named z, and we need to assign it a value 5, we use the following command
z<- 5
Similarly, the assignment operator =
is an alternative to <-
and is also used to assign values to variables.
z= 5
The right assignment operator assigns the value to a variable from right to left. It can be used in the following way in a command
5 -> z
Comparison Operators
We use comparison operators in R to compare values. These comparison operators include greater than “>” , less than “<“, greater than or equal to “>=”, less than or equal to “<=”, equal to “==” and Not equal to “!=”.
Now, if we want to check whether certain numbers or greater or less than others, we can use the following commands.
less <- 5 < 9 greater <- 12 > 20
Similarly, the comparison operators equal to and NOT equal to can be checked from the following command.
5==10
13!= 13
Here, the “==” sign shows equal to and “!=” sign shows not equal. Remember to use the signs correctly, or R will show an error for the command.
Logical Operators
The logical operators allow us to perform logical operations on logical values. The most basic logical operators are TRUE or just (T) and FALSE or just (F). These two are often used when it is required to check the validity of an object, i.e. if 10 is less than 3 or not. To check this in R, the following command is used
10<3
The output of the above command will be FALSE, because 10, by no doubt, is greater than 3.
Some of the other commonly used logical operators are Logical AND “&” Logical OR “|” Logical NOT “!”.
The logical operators including AND “&” OR “I”, and NOT “!” can be used to compare the values. The & operator used for logical AND operations returns TRUE if both conditions on the left and right are true. The |
operator is used for logical OR operations. It returns TRUE if at least one of the conditions on the left or right is true. Similarly, the ! operator is used for logical NOT operations. It negates a logical value, turning TRUE into FALSE and vice versa.
and <- 10 > 6 & 2 < 3 or <- 3 < 5 | 5 > 1 not <- !(2==3)
The output for the above commands will be following

We have explored the usage of different logical operations using simple numbers. What if we have a data frame containing numbers, and characters too? Let’s say we have the information of three students, including their student ID’s, age, GPA, and their pass or fail status, and we want to use logical operators on it for filtering and sorting data. To create this data set, we use the following command
student_data <- data.frame( StudentID = 1:3, Age = c(18, 20, 19), GPA = c(3.5, 3.2, 2.8), Pass = c(TRUE, TRUE, FALSE))
Once the above command is executed, the data frame has been created.
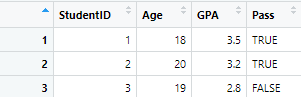
Now we perform different kind of logical operations and comparison operations on this data frame. Let’s say we just want to select the students that are above a certain age, i.e. 19 or above. To perform this operation, we use the following command
old_stud <- stu_data[stu_data$Age > 19, ] or old_stud <- stu_data[stu_data$Age >= 19, ]
The first command just selects the student having age above than 19, while the second command select students of the age equal to and above than 19. The output of the second command is shown as below
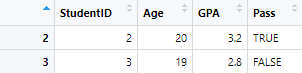
Similarly, if we want to filter out students based on their GPA, we can also use logical operators. For example, if only student of 3.5 GPA are required, we use the above data in a command in following way.

Multiple logical operators can also be used in a command, to filter multiple options from the data set. Let’s say we want to select students who have higher GPA and have passed the exams. We can filter these students using logical AND operator (&) to combine these conditions in a command, in the following way
students <- stu_data[stu_data$GPA >=3.5 & stu_data$Pass == TRUE, ]
By using the above command, student fulfilling the above requirement will be filtered only.
Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations in R. They include addition (+), subtraction (-), multiplication (*), division (/), exponentiation (^), modulus (%%), and integer division (%/%). We try these operators by using different examples.
Let’s say we have two objects by the name of x and y. We create these objects in R using following commands
x <- 15
y <- 9
The addition operator +
is used to add two numbers or concatenate vectors. So, for the above example, we add x and y by using following command
add <- x + y
Similarly, we carry out subtraction, multiplication, division, exponential and integer division by using the following commands.
subtract <- x - y multiple <- x * y division <- x / y exponent<- x ^ y integer<- x %/% y
The output for all these commands is given below, showing that arithmetic operations have been carried out correctly.
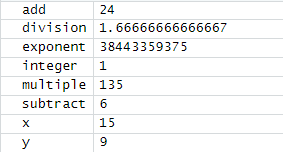
Miscellaneous Operators in R
There are other operators in R too, which are as helpful as arithmetic, assignment, comparison, and logical operators are. The colon or sequence operator “:” is used to generate a sequence of values from a starting point to an ending point. For instance, if we want to generate values from 1 to 5, following command should be used
seq<- 1:5
The concatenation operator “c” is used to combine multiple values into a vector. It is often used when creating vectors or lists. it can be used in a command in the following way
vec_a<- c(1, 2, 3)
or
vec_b <- c("patrick", "henry", "jack")
Similarly, there is another operator named subset operator in R. The subset operator “[ ]” is used to extract or subset elements from a data frame, vector, or list. For instance, if we create a vector and then want to extract certain elements from it as a subset, we can use the following command
vector <- c(10, 20, 30, 40, 50) subset <- vector[2:4]
First, the vector will be created having values as shown above, and then we use the index number of values that we want separately in a subset. The values from 20 to 40 will be selected in the subset, as shown in image below.

What if we want to select only a certain value in the subset, or more than one values not present in the sequence? This ca be done using the following commands
subset1 <- vector[4]
subset2 <- vector[c(1, 3)]
The above command will create two new vectors by the name of subset 1 and 2, having only the values for which we specified their index numbers. The result of above commands is shown below

Similarly, there is another operator named matrix multiplication operator. The “%*%” operator is used for matrix multiplication. It allows us to perform matrix multiplication between two matrices or between a matrix and a vector.
Let’s say we create two matrices, named X and Y by using the following command
X <- matrix(c(1, 2, 3, 4), nrow = 2) Y <- matrix(c(5, 6, 7, 8), nrow = 2)
And now we perform matrix multiplication by using the following command
Z<- X%*% Y
The following matrix is created by using the above command
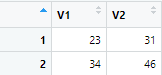
In conclusion, R offers a diverse range of operators, from arithmetic and assignment to comparison, logical, and specialized operators. We have discussed the important and useful operators in R, and by practicing them you can manipulate and analyze your data as per your requirements.